.NET development skills are in high demand these days, as they are useful for building a wide range of applications. If you're hoping to land a remote .NET developer job, you'll need to be prepared to answer some challenging questions from potential employers.
In this article, Ivan Baulin, Software Engineering Manager, and Aliaksei Harshkalep, Delivery Manager, who are also Certified .NET Technical Interviewers at EPAM Anywhere, have shared some examples of interview questions, which we have provided sample answers for. This will help you understand what to expect from .NET developer interviews and get ready.
1. What are the types of design patterns? Can you provide examples of their usage?
Design patterns are templates for solving common software design problems. They provide a way to structure code so that it is easy to maintain and extend.
There are three main types of design patterns:
- Creational Patterns: These patterns focus on object creation. Examples include the Singleton, Prototype, and Builder patterns.
- Structural Patterns: These patterns focus on how objects are composed to form larger structures. Examples include the Adapter, Decorator, and Façade patterns.
- Behavioral Patterns: These patterns focus on the communication between objects. Examples include the Observer, Mediator, and Visitor patterns.
2. What is REST API? Can you define it in your own words?
A REST API is a type of web service that uses HTTP requests to GET, PUT, POST, and DELETE data. A REST API can be used to access resources such as files, images, and other types of data.
3. Can you provide an example of a SOLID principle violation?
A SOLID principle violation would be if a class had too many responsibilities, making it difficult to maintain and change. For example, a class that both retrieves data from a database and saves data to a file would violate the Single Responsibility Principle.
Another example of a SOLID principle violation would be if a class wasn't sufficiently abstracted. This could make it difficult to extend the class or use it in different contexts. For example, a class that hard-codes values instead of using variables would violate the Open/Closed Principle.
Finally, an example of a SOLID principle violation would be if a class depended on another class that wasn't stable. This could lead to problems when the dependent class changes. For example, a class that depends on a library that's frequently updated would violate the Liskov Substitution Principle.
4. Can you describe the best CI/CD pipeline you can imagine?
There's no single answer to this question as it depends on the specific needs of a project. However, a good CI/CD pipeline typically includes the following features:
- Continuous integration: A process whereby code changes are automatically built and tested regularly. This helps to make sure that changes don't break the codebase and that new features work as expected.
- Continuous delivery: A process whereby code changes are automatically deployed to a staging or production environment. This helps to make sure that changes can be quickly and easily rolled out to users.
- Automated testing: A process whereby tests are automatically run against code changes. This helps to make sure that changes don't introduce bugs into the codebase.
- Code review: A process whereby code changes are reviewed by other developers. This helps to make sure that changes are correct and meet the standards of the team.
- Version control: A system that tracks changes to code over time. This helps to make sure that changes can be easily undone if necessary.
5. How would you address a growing technical debt?
There are a few ways to address technical debt:
- Refactor code: This involves making changes to the codebase to make it more readable, maintainable, and scalable.
- Add tests: This helps to make sure that changes don't introduce bugs into the codebase.
- Review code changes: This helps to make sure that changes are correct and meet the standards of the team.
- Use version control: This helps to make sure that changes can be easily undone if necessary.
- Implement automation: This helps to make sure that changes can be quickly and easily rolled out to users.
6. What is multithreading and why do we need it?
Multithreading is the ability of an operating system to execute multiple threads concurrently. Threads are similar to processes, but they're lighter and share the same address space. Multithreading enables your application to keep the UI responsive while performing long-running tasks in the background.
We need multithreading because:
- It allows us to utilize idle CPU time more efficiently.
- It makes our applications more responsive to user input.
- It allows us to perform multiple tasks simultaneously.
- It helps us avoid race conditions and deadlocks.
7. When do you use asynchronous methods?
You use asynchronous methods to improve the responsiveness of your application. Asynchronous methods are particularly useful when you are working with I/O bound operations, such as accessing a database or a web service over the network.
Calling an asynchronous method doesn't block the current thread. Instead, the current thread is free to do other work while the asynchronous method is running in the background. When the asynchronous method completes, it can notify the original thread that it is finished, at which point that thread can resume execution.
One common scenario where asynchronous methods are used is when implementing a user interface. For example, if you have a button in your UI that starts a long-running operation, such as downloading a file from a web server, it can be beneficial to execute that operation asynchronously. That way, the button can remain responsive so that the user can click it again or perform other actions, and the UI can continue to update itself based on changes in the application state.
8. What are the conditions for garbage collection?
Garbage collection in .NET occurs when an object is no longer being used by the application and there's no reference to that object. The garbage collector will automatically clean up these objects and free up the memory that they are occupying.
Garbage collection is an automatic process and developers don't have to write any code to explicitly mark objects for garbage collection.
9. What is LINQ?
C# and Visual Basic now include LINQ (Language Integrated Query), which is a pre-existing set of capabilities introduced in Visual Studio 2008 that extends strong query abilities to the language syntax. The technology may be expanded to support any sort of data store, and there are standard, easily-learned patterns for querying and updating data.
You can use LINQ queries in C# or Visual Basic to filter, identify, and project data from arrays, collections, XML documents, relational databases, and other third-party data sources. LINQ helps to bridge the gap between data and objects.
10. What is inheritance in .NET?
Inheritance is one of the key features of object-oriented programming (OOP) that allows you to create relationships between classes. It enables you to create a new class (derived class) from an existing class (base class). The derived class inherits all the members of the base class, but it can also add its own members.
There are two types of inheritance in .NET:
- Single inheritance is when a derived class inherits from only one base class.
- Multiple inheritances is when a derived class inherits from more than one base class.
11. What are commonly used .NET collection types?
There are many ways to collect data, but the most popular options in modern applications are hash tables and queues. They both provide fast indexing with reasonable space usage for large datasets.
If your program needs more flexibility than these two database types offer, then it may be worth exploring other options, like stacks or bags, where each element can act as its own container rather than being allocated pre-sized space, as in arrays.
- ArrayList is a dynamic array that can grow and shrink as needed. It doesn't have a fixed size, so you can add or remove items from it.
- Queue is a first-in, first-out (FIFO) data structure that can only contain objects of the same type.
- Stack is a last-in, first-out (LIFO) data structure that can only contain objects of the same type.
Some of the other commonly used .NET collection types are:
- Hashtable
- SortedList
Each of these collection types has its own advantages and disadvantages that you should consider before using them in your applications.
A practical .NET coding task
You have a simple class with a set of properties. You need to override the Equals() method to have value-like behavior (if all properties are equal, then objects are equal):
This is a simple task; however, it allows the interviewer to check a lot of things:
- Generic ability to use C# syntax
- Ability to write safe code, for example, check null values, and safe type casting
- Absence of redundant statements, like lots of if/else or try/catch syntax
- Knowledge of modern C# syntax, for example, that changing class to record would automatically give you the desired behavior
- Whether you can follow up on GetHashCode() function details and how it's related to Equals()
How to nail interview questions for .NET developers
When you're interviewing for a .NET developer role, the interviewer is looking to see if you have the necessary technical skills and cultural fit for the team. There are a few key things to keep in mind when preparing for your interview:
- Do your research: Read up on the company culture and values, as well as the specific technologies used in the role you're interviewing for. This will help you answer questions about why you're interested in the position and how your skills fit with the company's needs.
- Be prepared to code: Often, you'll be asked to write code during your interview. Be prepared with a laptop so that you can quickly jump into a coding exercise.
- Check your internet access: For a remote role, having a strong and reliable internet connection is key. Make sure you have a backup plan in place, just in case your primary internet connection goes down.
- Have questions ready: Asking thoughtful questions shows that you're truly interested in the role and the company. Prepare a few questions ahead of time so that you're not caught off guard during the interview.
- Practice, practice, practice: In addition to coding exercises, you should be prepared to answer questions about your resume and experience. Be ready to discuss your skill set in detail, and have examples of previous projects or challenges you've tackled.
Finally, relax. You're at the interview stage because they like what they've seen so far — now it's just a matter of getting to know you better and seeing if you're a good fit for the team.
We thank Ivan Baulin, Software Engineering Manager, and Aliaksei Harshkalep, Delivery Manager, for contributing to this article.
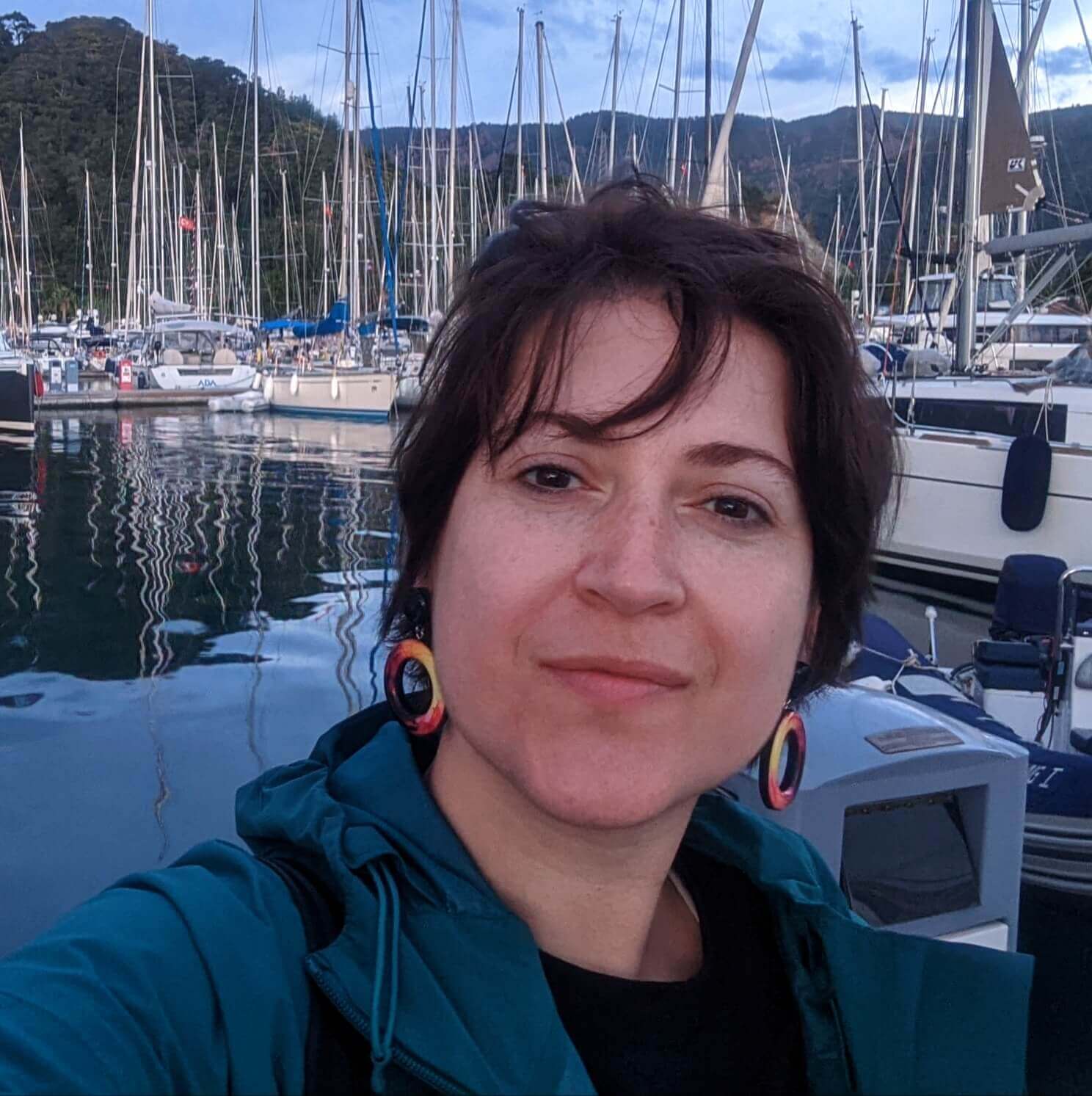
As Chief Editor, Darya works with our top technical and career experts at EPAM Anywhere to share their insights with our global audience. With 12+ years in digital communications, she’s happy to help job seekers make the best of remote work opportunities and build a fulfilling career in tech.
As Chief Editor, Darya works with our top technical and career experts at EPAM Anywhere to share their insights with our global audience. With 12+ years in digital communications, she’s happy to help job seekers make the best of remote work opportunities and build a fulfilling career in tech.
Explore our Editorial Policy to learn more about our standards for content creation.
read more